The Design of Carry-lookahead Adder and Subtractor-數位邏輯技術
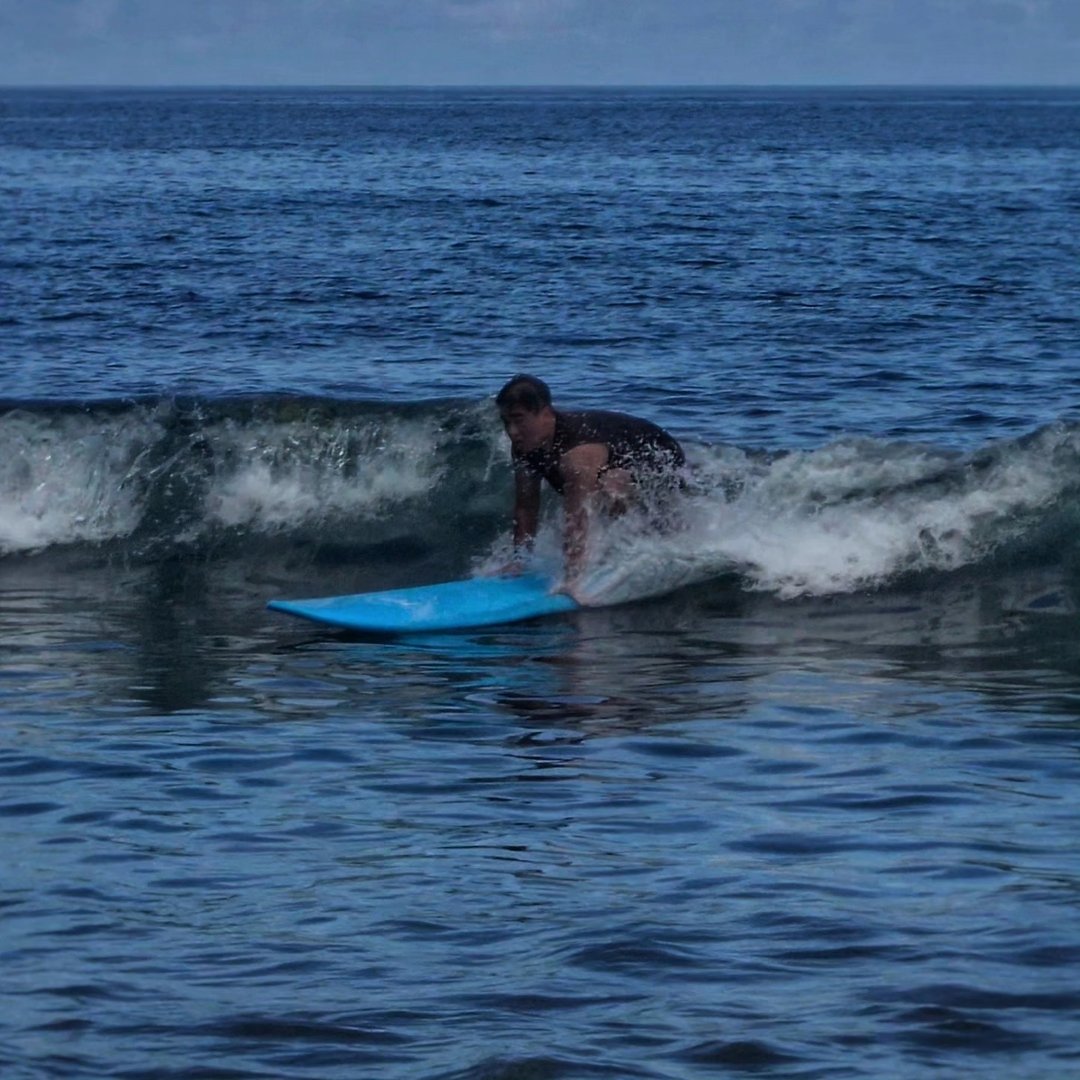
The Design of Carry-lookahead Adder and Subtractor
Introduction to Carry-lookahead Adder and Subtractor
References
Simple Explanation
- Basically, the main idea of carry-lookahead adder is doing the
calculation of carry before doing the addition.
- To do so, we have to generate both
(propagate) terms and (Generate) terms. - After the calculation of all carrys, we have to do the
summation/addition.
- The final step is to proccess the result.
- To check if overflow occurs:
- The sign of the result: the last bit of the result equals to its sign.
- The value of carry_out: the last bit of carry is the value of carry_out.
- To check if overflow occurs:
- To do so, we have to generate both
The VHDL code of Carry-lookahead Adder
Carry-lookahead Adder
1 | library ieee; |
Carry-looahead Adder with two sign control and application of 7-seg Decoder
1 | library ieee; |
Source Code
- Title: The Design of Carry-lookahead Adder and Subtractor-數位邏輯技術
- Author: Shih Jiun Lin
- Created at : 2023-10-04 22:00:00
- Updated at : 2023-10-08 00:33:31
- Link: https://shih-jiun-lin.github.io/2023/10/04/The Design of Carry-lookahead Adder and Subtractor-數位邏輯技術/
- License: This work is licensed under CC BY-NC-SA 4.0.