Arrays, Strings and Vectors-程式設計筆記
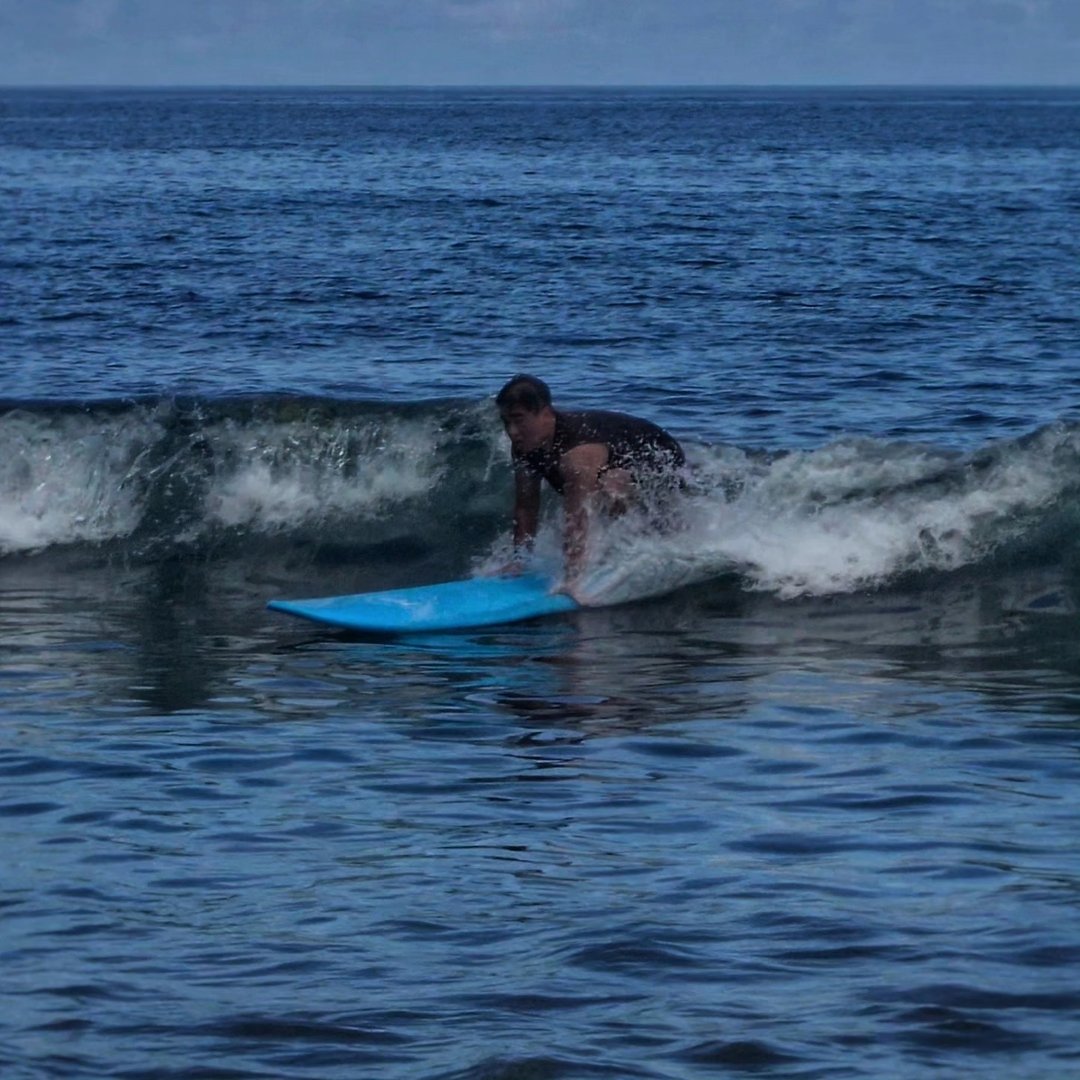
Chapter 6 Arrays, Strings and Vectors
Array
- If there are not enough initializer for a given array, the remaining
elements will be initialized to 0.
1
2
3
4
5
6
7
8
9
10
11
12
13
int main(){
int a[]={0, 1, 2, 3, 4};
// the compiler will automatically recognize it as a[4]
int b[4]={0, 1};
// b[0]=0, b[1]=1
//b[2]~b[4]=0
int c[4];
//The value c[0]~c[4] will be random
int x;
cin>>x;
int d[x];
//It's not allowed1
2
3
4
5
6
7
8
9
10
11
12
13
int main(){
int x{0};
std::cin>>x;
int a[x];
for(int i=0; i<=x; i++){
a[i]=i;
}
for(int j=0; j<=x; j++){
std::cout<<a[j]<<" ";
}
}
// It's allowed
Using '==' with arrays is not allowed
- Using '==' to compare an array with another is not allowed, since the "array_name" is the address of the first element in array_name[];
Input a array to a function
- Entire array -> passed by reference
- Individual array elements -> passed by value.
- By adding const in function, the entire array won't be changed.
example(1D by value):
1 |
|
example(1D by reference with pointer):
1 |
|
example (2D by value):
1 |
|
example(2D by reference with pointer):
1 |
|
Multidimensional Array
- If there are not enough initializer for a given array, the remaining
elements will be initialized to 0. Ex:
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
using namespace std;
int main(){
int array[2][3]={{0, 1, 2}, {3, 4, 5}};
for(int i=0; i<2; i++){
for(int j=0; j<3; j++){
cout<<array[i][j]<<" "; //0 1 2
//3 4 5
}
cout<<"\n";
}
int array2[2][3]={{0, 1}, {3}};
for(int i=0; i<2; i++){
for(int j=0; j<3; j++){
cout<<array2[i][j]<<" "; //0 1 0
//3 0 0
}
cout<<"\n";
}
}
//If the number isn't being input, the element will be 0. - Passing a multidimensional array to a function
1
2
3
4
5
6void function_2D(const int a[][nums]){
...
}
void fucntion_3D(const int a[][nums][nums]){
...
}
Calculating the address of an array.
- A 2D array with 6 elements, if the address of
is 100, what is the address of ? =116, since an address will occupied 4 bytes.
- A 3D array with 24 elements, if the address of
is 200, waht is the address of and ? =280 =244
Char Array and String(C#)
Reference: link
- Char array
- One char is 1 byte.
- Declaration:char char_name[arraysize];
- String
#include
cstring the size of the string is chars+1 bytes.
- ex: dog => 4 bytes
c_string must be ended with '\0'.
initialization:
1
2
3
4
5
6
7
using namespace std;
int main(){
char a[10]={'H', 'e', 'l', 'l', 'o', 'w', 'o', 'r', 'l', 'd'}; //by using {}, the size of the array must be word_amount;
char b[11]="Helloworld"; //by using "", the size of a array must be word_amount+1;
cout<<a<<" "<<b;
}cin.getline(char_name, char_length, end_condition)
1
2
3
4
5
6
7
8
using namespace std;
int main(){
char string[11];
cin.getline(string, 11, '\n');
cout<<string;
}'\0'!='0'.
One Chinese character = 2 bytes.
strcpy(str1, str2) => copy string2 to string 1.
strcat(str1, str2) => str1 + str2
strcmp(str1, str2) => compare str1 and str2.
ex:
1
2
3
4
5
6
7
8
9
using namespace std;
int main(){
char a[50], b[50];
strcpy(a, "Hi hello world.");
strcpy(b, "Hi hello");
cout<<strcat(a, b);
}
String (C++)
References:link
- #include
string - declaration:
1
2
3
4
5
6
7
int main(){
string s="Hi";
string s("Hi");
//both of them are the same.
} - input variables
- with getline(), we can input strings with spaces.
1
2
3
4
5
6
7
using namespace std;
int main(){
string s;
getline(cin, s);
cout<<s;
}
- with getline(), we can input strings with spaces.
- Using '+' with strings is allowed
1
2
3
4
5
6
7
using namespace std;
int main(){
string a="Hello";
string b="World";
cout<<a+b; //cout HelloWorld
} - Using "==" and "!=" to compare two strings is allowed
1
2
3
4
5
6
7
8
9
10
11
12
13
14
using namespace std;
int main(){
string s1, s2;
getline(cin, s1);
getline(cin, s2);
if(s1==s2){
cout<<"same";
}
else{
cout<<"different";
}
} - Creating copy of strings
- str1=str2 => str1(str2).
- at()
- str[1]=> str
at(1).
- str[1]=> str
- length()
- Gives u the length of a string.
- empty()
- return true if the string is empty.
- substr( )
- return a specific part of a string. substr(a, b) => return the
part of the string bewteen
a, b . 1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
using namespace std;
int main(){
string test;
test="Hello World";
cout<<test.substr(0, 5);
}
```
## Linear search
* To find the corresponding element of a value;
Example:
```c++=
void search(int a[], int value, int arraysize){
int flag=0, element;
for(int i=0; i<arraysize; i++){
if(value==a[i]){
flag=1;
element=i;
}
}
if(flag==1){
std::cout<<"The corresponding element of the value is: "<<element<<'\n';
}
else{
std::cout<<"There is no corresponding element to your value.\n";
}
}
int main(){
int a[5]={1, 2, 3, 4, 5};
int value;
while(std::cin>>value){
search(a, value, 5);
}
}
- return a specific part of a string. substr(a, b) => return the
part of the string bewteen
Bubble Sort
Reference:link
1 |
|
Vectors
Reference: link
- Title: Arrays, Strings and Vectors-程式設計筆記
- Author: Shih Jiun Lin
- Created at : 2023-01-17 23:00:50
- Updated at : 2023-01-24 01:32:12
- Link: https://shih-jiun-lin.github.io/2023/01/17/Chapter 6 Arrays, Strings and Vectors/
- License: This work is licensed under CC BY-NC-SA 4.0.