Functions and Introduction to Recursion-程式設計筆記
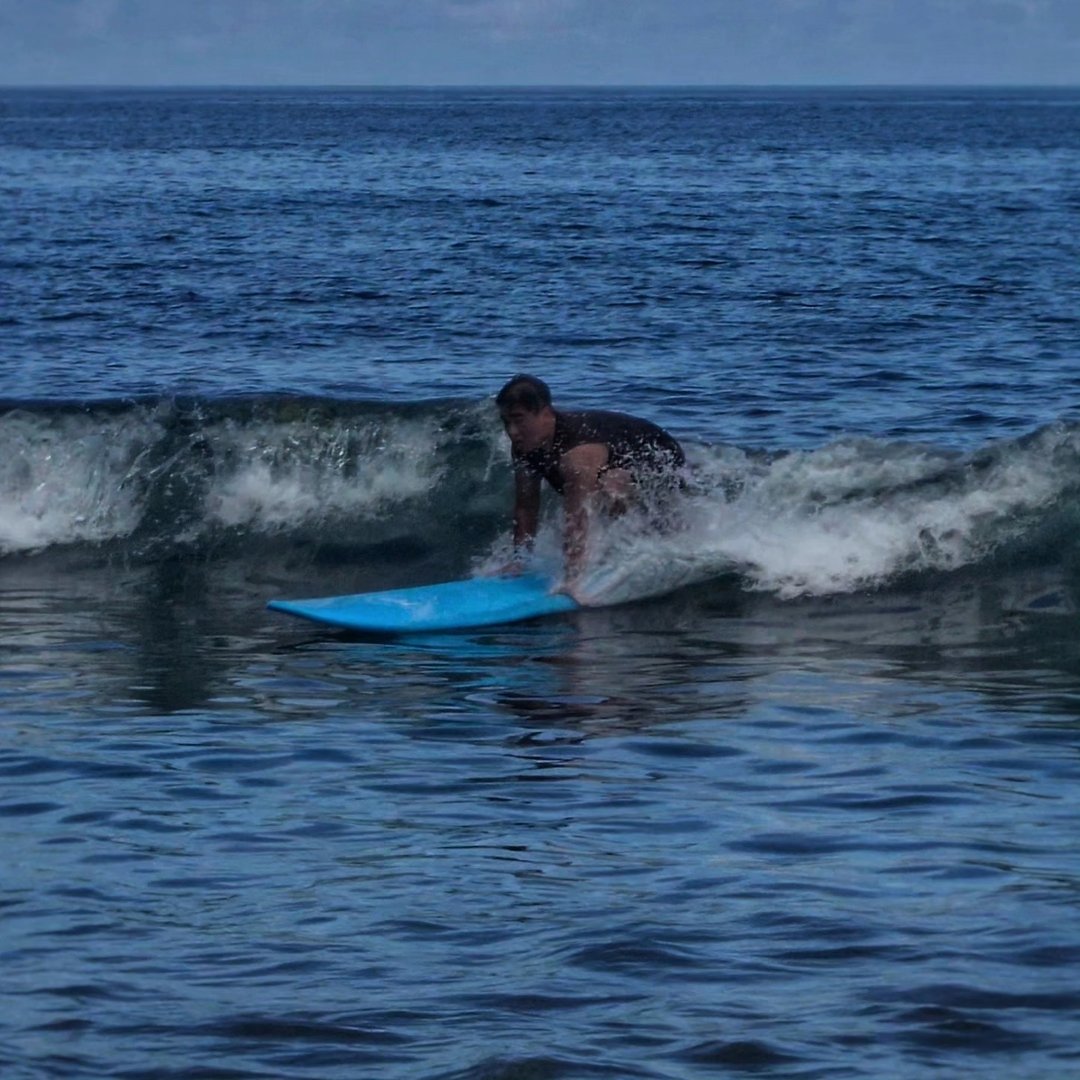
Chapter 5 Functions and Introduction to Recursion
Cmath
1 |


Defining Our Own Fucntion
- Exapmple(square function)
1
2
3
4
5
6
7
8
int square(int); //function prototype declaration
int main(){
...sth
}
int square(int y){
return y*y;
} - We can define a function with default value.
- Default value must be the rightmost in a function’s parameter list.
1 |
|
void
- Used when defining a function that doesn't return a value.
1
2
3void square(int x){
std::cout<<x*x;
}
Standard Library Header Files
Random Number
1 |
|
Time Function
1 |
|
Why is rand() not that random?
- You have to set random seed.
1
2
3
4
5
...
unsigned int seed{0};
cin>>seed; //input a random number.
srand(seed);1
2
3
4
5
...
srand(time());
...
Enumerate
- If i set win=1, lost will be 2, continue will be 3.
- win{1} is not allowed, only win=1 will work.
1
2
3//example
enum Game_Status{WIN, LOST, CONTINUE};
//win=0, lost=1, continue=2.
static using with local variables
- You can see it as a way to make local variable to become a global variable.
- It will only be initialized one time!
1
2//example
static int x=1;1
2
3
4
5
6
7
8
9
10
11
12
13//Example code
using namespace std;
void static_num(){
static int x=1;
cout<<x<<"\n";
x++;
cout<<x<<"\n";
}
int main(){
static_num();
static_num();
}
static using with global variables
- The variable used with static is only accessible for the specific file.
extern
- Get a variable from another file. Ex:
1
2//file_1
int a=1;1
2//file_2
extern a;
inline
- The cost of defining a function is too big,inline will decrease the cost.
- The concept of inline is expansion
- inline can only be used when doing simple calculation.
1
2
3
4
5
6
7
8
9
10
using namespace std;
inline cube(int x){
return x*x*x;
}
int main(){
int x=0;
cin>>x;
cout<<cube(x); //cube(x)=x*x*x
}
Pass by reference.
- It will affect the original value of a variable.
- Adding a '&' in front of a variable, with '&' the function will directly put the variable (num) into the function.
- It won't use extra ram space to store a number. (int a=1; int b=a; will use extra ram space)
- The concept is like domain.
1
2
3void function(int &number){
...
}1
2
3
4
5
6
7
8
9
10
11
12//example
using namespace std;
void cube(int &x){
x=x*x*x;
}
int main(){
int num=0;
cin>>num;
cube(num);
cout<<num;
}
Acces to a global variable
1 |
|
Function overloading
- C++ enables several functions of the same name to be defined, as long as they have different signatures.
- The compiler will automatically choose the proper function to
execute. Depending on the data type or the amount of the parameter
list
1
2
3
4
5
6
7
8
9
10
11
void square(int x){
std::cout<<x*x<<"\n";
}
void square(double x){
std::cout<<x*x<<"\n";
}
int main(){
square(1); //cout 1
square(1.1); //cout 1.21
}
Making and using your own library
- Example: Finding max of three numbers.
- .h template
- template <class T> means that every type of data can use this function.
- The way to decalre a template function is the same as normal
function.
1
2
3
4
5
6
7
8
9
10
11template <class T>
T maximum(T value1, T Value2, T Value3){
T maximumValue =value1;
if(Value2>maximumValue){
maximumValue=Value2;
}
if(Value3>maximumValue){
maximumValue=Value3;
}
return maximumValue;
} - main program
1
2
3
4
5
6
7
8
int main(){
int x, y, z;
while(std::cin>>x>>y>>z){
std::cout<<"Max= "<<maximum(x, y, z);
}
}
Recursion
- A function calling oneself.
- Example of factorial of a nonnegative function with recursion.
1
2
3
4
5
6
7
8
9
unsigned long factorial(unsigned long num){
if(num<=1){
return 1;
}
else{
return num*factorial(num-1);
}
} - Example of Fibonacci Series
1
2
3
4
5
6
7
8
9
unsigned long fib(usigned long num){
if(num==1||num==0){
return 0;
}
else{
return fib(num-1)+fib(num-2);
}
}
- Title: Functions and Introduction to Recursion-程式設計筆記
- Author: Shih Jiun Lin
- Created at : 2023-01-17 23:00:50
- Updated at : 2023-01-24 01:32:02
- Link: https://shih-jiun-lin.github.io/2023/01/17/Chapter 5 Functions and Introduction to Recursion/
- License: This work is licensed under CC BY-NC-SA 4.0.