Struct and Class-程式設計筆記
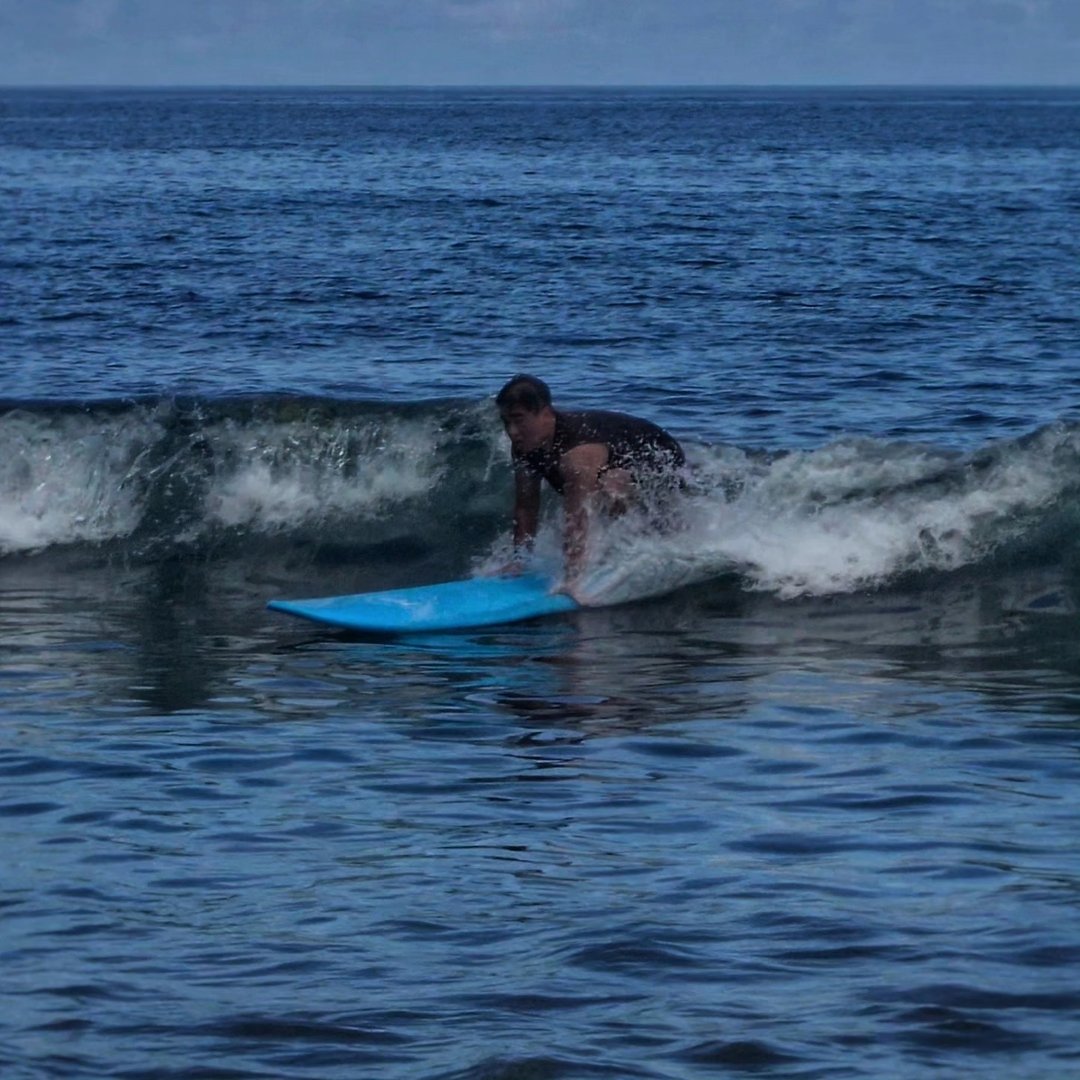
Chaper 9 Struct and Class
Class
What is class?
- Class is a template with Data members and Methods. A instance generated by a template is called an "object".
public, private and protected Reference:link
- Public => Accessible to everone.
- Private => Only accessible to the "Class".
- Protected => Only accessible to the "class" and its "subclass".
".", "->" and "::"
Reference: link
Ex1:
1 |
|
Ex2:
1 |
|
- Writing files separately
Ex:
"Say_sth.h":
1 |
|
Say_sth.cpp:
1 |
|
Say_sth_main.cpp:
1 |
|
Result:
Constructor and Destructor Reference: link
Constant and Class
- To specify an object is not modifiable.
- A const member function is specified as const bothin its prototype and in its definition.
Composition: Objects as Members of Classes Reference:link
- The constructor of one class will automatically create a default constructor(default copy constructor) to copy an object when needed.
Ex:
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
48
49
50
51
52
53
54
using namespace std;
class Name{
public:
Name(string fn="F_Name", string ln="L_name");
void setName(string f_name, string l_name);
void const output();
private:
string f_name;
string l_name;
};
Name::Name(string fn, string ln)
:f_name(fn),l_name(ln)
{
}
void Name::setName(string f_name, string l_name){
this->f_name=f_name;
this->l_name=l_name;
}
void const Name::output(){
cout<<f_name<<" "<<l_name;
}
class Say_sth{
public:
Say_sth(Name &name, string w="Helloworld");
void input(Name &name, string words);
void const output();
private:
Name test;
string words;
};
Say_sth::Say_sth(Name &name, string w)
:test(name),words(w)
{
}
void Say_sth::input(Name &name, string words){
this->test=name;
this->words=words;
}
void const Say_sth::output(){
test.output();
cout<<" said "<<words<<"\n";
}
int main(){
Name name, name2;
name.setName("Ryan", "Lin");
name2.setName("Bryan", "Lin");
Say_sth say(name);
say.output();
say.input(name2,"HaHaPiyan");
say.output();
}Initializer(Constructor)
Ex:
Friend Function
- Defined outside public or private.
- Have the access to public and private members.
Ex:
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
using namespace std;
class Say_sth{
friend void input(Say_sth &say_sth, string name, string words);
public:
Say_sth(string n="Name", string w="Helloworld");
void const output();
private:
string name;
string words;
};
Say_sth::Say_sth(string n, string w)
:name(n),
words(w)
{
}
void input(Say_sth &say_sth, string n, string w){
say_sth.name=n;
say_sth.words=w;
}
void const Say_sth::output(){
cout<<name<<" said: "<<words<<"\n";
}
int main(){
Say_sth test;
input(test, "Ryan", "HaHaPiyan");
test.output();
}Static Member Function/Data
- A static can only access to a static data.
1 |
|
Result:
this->
- Usually used when one variable's name is the same as the member of a class.
- The difference between return *this and return this.
Ex1(same name):
Ex2(cascaded member-function called):
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
using namespace std;
class Say_sth{
public:
Say_sth(string n="Name", string w="Helloworld");
Say_sth &setName(string name);
Say_sth &setWords(string words);
void const output();
private:
string name;
string words;
static int count;
};
Say_sth::Say_sth(string n, string w)
:name(n),
words(w)
{
}
Say_sth &Say_sth::setName(string name){
this->name=name;
return *this;
}
Say_sth &Say_sth::setWords(string words){
this->words=words;
return *this;
}
void const Say_sth::output(){
cout<<name<<" said: "<<words<<"\n";
}
int main(){
Say_sth test;
string name, words;
while(getline(cin,name)){
getline(cin,words);
test.setName(name).setWords(words);
test.output();
}
}Ex3:
Result:1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
using namespace std;
class Say_sth{
public:
Say_sth(string n="Name", string w="Helloworld");
void input(string name, string words);
void const output();
private:
string name;
string words;
};
Say_sth::Say_sth(string n, string w)
:name(n),
words(w)
{
}
void Say_sth::input(string name, string words){
this->name=name;
this->words=words;
}
void const Say_sth::output(){
cout<<name<<" said: "<<words<<"\n";
cout<<this->name<<" said: "<<this->words<<"\n";
cout<<(*this).name<<" said: "<<(*this).words<<"\n";
//The code above are the same.
}
int main(){
Say_sth test;
test.input("Ryan", "HaHaPiyan");
test.output();
}Operator Overloading
- Operators that can be overloaded
- Operators that cannot be overloaded
Ex:
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
using namespace std;
class Complex{
public:
Complex(double real=0, double img=0);
Complex operator+(const Complex &a);
Complex operator-(const Complex &a);
void Display();
private:
double Real;
double Img;
};
Complex::Complex(double real, double img)
:Real(real),Img(img)
{
}
Complex Complex::operator+(const Complex &a){
return Complex(Real+a.Real, Img+a.Img);
}
Complex Complex::operator-(const Complex &a){
return Complex(Real-a.Real, Img-a.Img);
}
void Complex::Display(){
cout<<"Real: "<<Real<<"\n";
cout<<"Img: "<<Img<<"\n";
}
int main(){
Complex a(1, 2), b(3, 4), c;
c=a+b;
c.Display();
c=a-b;
c.Display();
}- Operators that can be overloaded
Struct
- Basic Application
Ex:
1 |
|
- Pointers for a Structure
Ex:
1 |
|
- Title: Struct and Class-程式設計筆記
- Author: Shih Jiun Lin
- Created at : 2023-01-17 23:00:50
- Updated at : 2023-01-24 01:30:57
- Link: https://shih-jiun-lin.github.io/2023/01/17/Chaper 9 Struct, Class/
- License: This work is licensed under CC BY-NC-SA 4.0.