Pointers-程式設計筆記
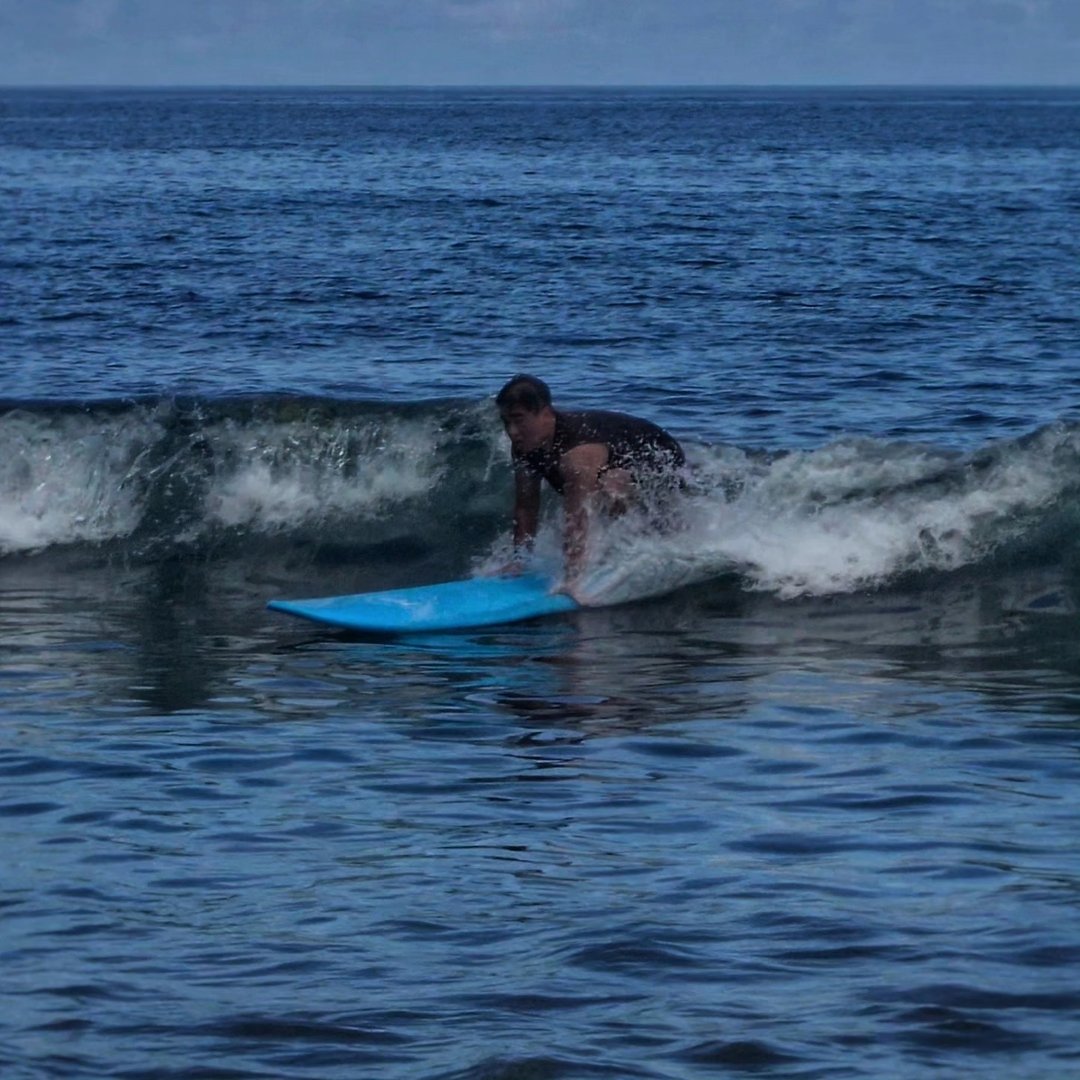
Chaper 7 Pointers
What is Pointers?
- Declaration: int pointer_name;/char pointer_name;
- Data being stored inside a pointer is the address(unsigned int: hex) of a data in the memory.
- Pointer shoud be initialized. A pointer with null or the value 0 is called null pointer.(0 is the only integer that can be assigned to a pointer variable)
ex:
How to assign the address of a data to pointers?
- "*" and "&" are inverses of one another. ex:
1
2
3
4
5
6
7
8
9
10
11
using namespace std;
int main(){
int *pointer;
int a=10;
pointer=&a;
cout<<pointer<<"\n"; //cout the address of a.
cout<<*pointer<<"\n";cout<<the value of a.
cout<<*&a; //cout the value of a.
}
The relations between pointers and data
- alter a <=> alter the value of *pointer.
- The address won't be changed.
ex:
1 |
|
PassByReference with Pointers
ex:
1 |
|
Const and Pointers
1 | const int* pointer1=# //The number pointer1 points to is a const. |
- For pointer1:
- For pointer2:
ex(For pointer1):
1 |
|
ex(For pointer2):
1 |
|
Pointers and Array
int array and pointers
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
using namespace std;
int main(){
int a[5]={0, 1, 2, 3, 4};
int *pointer=a; //a is the address of a[0]
for(int i=0; i<5; i++){
cout<<*(a+i)<<" "; //cout 0~4
}
cout<<endl;
for(int i=0; i<5; i++){
*(a+i)=0;
}
for(int i=0; i<5; i++){
cout<<*(a+i)<<" "; //cout 0
}
cout<<endl;
cout<<&a[4]-pointer; //4 the distance(elements) between a[4] and pointer(a[0]). = 2*sizeof(int)
}char array and pointers
1
2
3
4
5
6
7
8
9
10
using namespace std;
int main(){
char a[18]="Helloworld";
cout<<a<<"\n"; //it won't cout the address of a[0]
cout<<a+5<<"\n"; //it will cout world
cout<<*(a+9)<<"\n"; //equals to a[10];
cout<<(void*)a<<"\n"; //it will cout the address of a[0](H)
cout<<(void*)a+9<<"\n"; //it will cout the address of a[9](d)
}c-string and pointers
sizeof()
- sizeof() with array or value will return the total size in bytes.
Ex1(with array):
1 |
|
Ex2(with value):
1 |
|
Ex3(with c-string):
1 |
|
- void* => a pointer for any genre of data type.
- Before using it, the type of pointer must be determined.
1 |
|
- Use pointers to print a 2d array
1 |
|
- Pointers for 2D array
- Pointers for 3D array
Arrays of Pointers
- The array stores the address of the first character in each c-string.
Ex1:
1
2
3const char * const suit[ 4 ] =
{ "Hearts", "Diamonds",
"Clubs", "Spades" };Ex2:
1
2
3
4
5
6
7
8
using namespace std;
int main(){
char *suit[2]={"Hello", "World"}; //stores the address of the first character in each string
for(int i=0; i<sizeof(suit)/8; i++){
cout<<suit[i]<<" ";
}
}
Function's Pointer
- Pass the pointer of a function to another fucntion
1 |
|
Selection sort
Reference(How it works):link
- Easy to program but inefficient. Ex:
1 |
|
- Title: Pointers-程式設計筆記
- Author: Shih Jiun Lin
- Created at : 2023-01-17 23:00:50
- Updated at : 2023-01-23 22:38:45
- Link: https://shih-jiun-lin.github.io/2023/01/17/Chaper 7 Pointers/
- License: This work is licensed under CC BY-NC-SA 4.0.